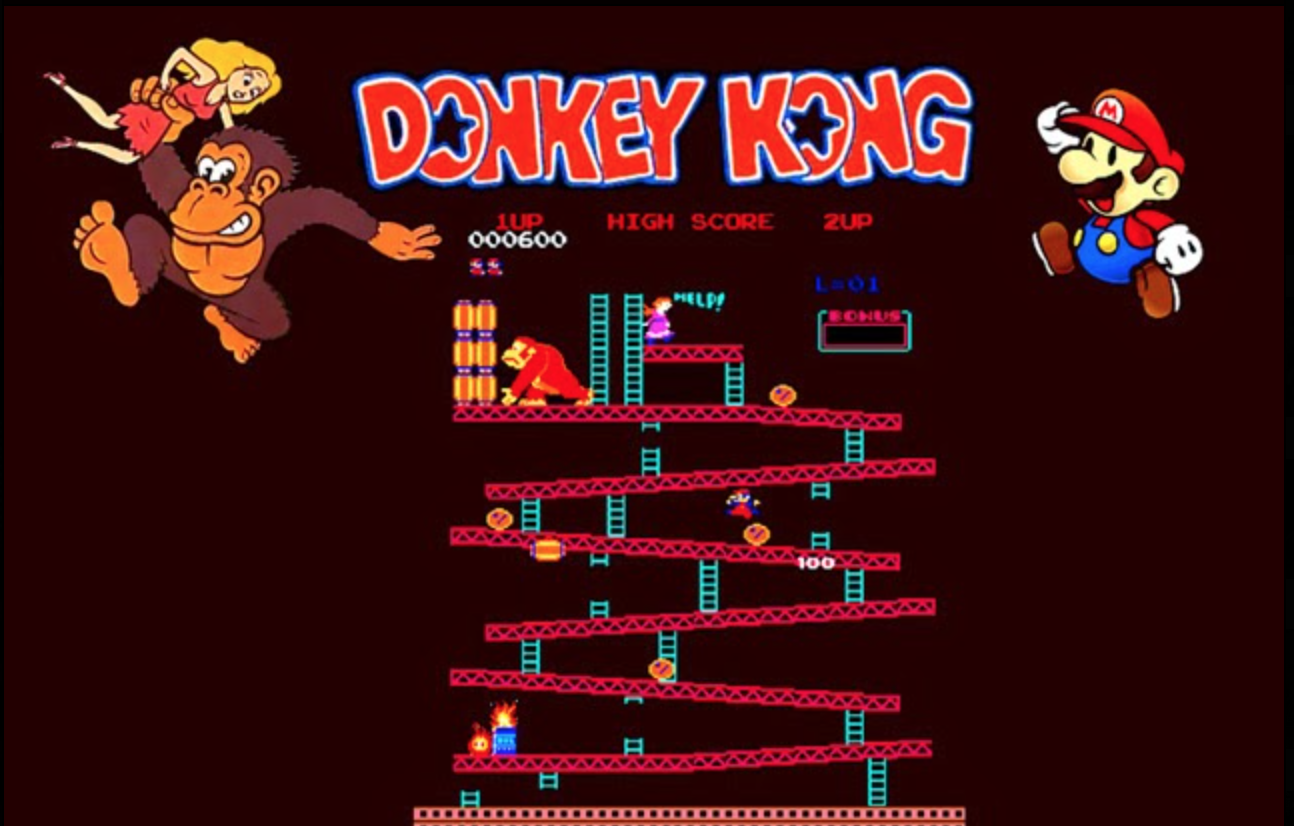
Goal: Emulate a modified version of the original 1981 Donkey Kong on the FPGA board by integrating RTL and System-on-Chip (SoC) with USB and VGA Interface.
Block Diagram
Task completed to finish the project:
Create Sprites: Convert PNG images to hex values by mapping each pixel in the image to a specific hex value specified by the color palette. This allowed me to use 16 colors for the entire game.
Animation: Sprite animations were achieved by cycling through multiple sprites within a specific count. For example, when the left directional key is pressed, Jumpman runs to the left with animations using a counter that is only incremented when the key is pressed. As the counter cycles, a specific sprite would be drawn for a given range of the counter.
Jumpman Movements: Jumpman’s movements were determined by key presses from the USB keyboard.
Collision Detection: Collision detection was implemented by marking a region in each barrel and if that region crosses Jumpman position then a collision signal goes high
Angled Platforms and Background Detection: The platform on which Jumpman stands is not always a constant height thus I had to come up with a way to make him rise up when he gets to the next step. My solution was to make a fake background that marks each step(white vertical lines) and when Jumpman crosses the marker his direction in the y-axis is decremented by a pixel. Going down the platform is handled by gravity. A similar method was also implemented for going up ladders and going down ladders. When up is pressed, Jumpman can only go up if he is in front of the teal color and he can only go down a ladder if his foot is standing on dark blue. If his foot is on the color black then gravity takes effect. Jumpman movements effectively were governed by the fake background but the real background was the one drawn.
Barrel Movement/Regeneration: Four barrels were created. Two, which were only affected by gravity, and two which only went down ladders. For the barrels that were only controlled by gravity, the x motion was increment by one and bounce off the wall when it hit the border. The other two went down the ladder when the pixel underneath them was dark blue. When the barrels got to the oil can their motion stopped and the draw flag for the barrel goes low so the barrel disappear but when Donkey Kong wants the barrel, it gets respawned at Donkey Kong's hand and the draw flag goes high again.
Code: System Verilog was used to create the game. C++ was used for the USB keyboard protocol. A snippet of one module is shown below.
The “Jman_Movement” code took inputs from the keyboard and updated the position of Mario as he traversed the map.
Jman_Movement( input Clk, input logic frame_clk, input logic w_on, a_on, s_on, d_on , output sprite_to_Draw, output logic[9:0] j_start_x output logic[9:0] j_start_y ); parameter [9:0]shape_x = 8'd379 ; parameter [9:0]shape_y = 10'd395 ; parameter [9:0] jman_X_Min = 10'd0; parameter [9:0] jman_X_Max = 10'd639; parameter [9:0] jman_Y_Min = 10'd0; parameter [9:0] jman_Y_Max = 10'd479; parameter [9:0] X_Step = 10'd5; parameter [9:0] Y_Step = 10'd5; logic [9:0] jman_X_Pos, jman_X_Motion, jman_Y_Pos, jman_Y_Motion; logic [9:0] jman_X_Pos_in, jman_X_Motion_in, jman_Y_Pos_in, jman_Y_Motion_in; logic [3:0] counter ; logic [3:0] counter_in ; logic [4:0]sprite_to_Draw ; logic [4:0]sprite_to_Draw_next; logic [4:0]last_sprite; logic [4:0]last_sprite_next; assign [9:0]j_start_x = jman_X_Pos ; assign [9:0]j_start_y = jman_Y_Pos; logic frame_clk_delayed, frame_clk_rising_edge; always_ff @ (posedge Clk) begin frame_clk_delayed <= frame_clk; frame_clk_rising_edge <= (frame_clk == 1'b1) && (frame_clk_delayed == 1'b0); end always_ff @ (posedge Clk) begin if (Reset) begin jman_X_Pos <= shape_x; jman_Y_Pos <= shape_y; jman_X_Motion <= 10'd0; jman_Y_Motion <= 10'd0; sprite_to_Draw <= 5'd01; last_sprite <= 5'd01; end else begin jman_X_Pos <= jman_X_Pos_in; jman_Y_Pos <= jman_Y_Pos_in; jman_X_Motion <= jman_X_Motion_in; jman_Y_Motion <= jman_Y_Motion_in; sprite_to_Draw<= sprite_to_Draw_next; last_sprite <= last_sprite_next; end end always_ff @(posedge(frame_clk_rising_edge)) begin if(Reset) begin counter <= 4'b0; counter_in <= 4'b0; end else counter<= counter_in; end always_comb begin // By default, keep motion and position unchanged jman_X_Pos_in = jman_X_Pos; jman_Y_Pos_in = jman_Y_Pos; jman_X_Motion_in = jman_X_Motion; jman_Y_Motion_in = jman_Y_Motion; sprite_to_Draw_next = sprite_to_Draw; last_sprite_next = last_sprite; unique case(Keycode) // up_on: left_on: jman_X_Motion_in = ~(X_step) + 1'b1; last_sprite_next = 5'd06 ; if(counter[3] == 1'b1) sprite_to_Draw_next = 5'd07 ; else begin sprite_to_Draw_next = 5'd08 ; end counter_in = counter +1'b1; // down_on: right_on: jman_X_Motion_in = X_step; last_sprite_next = 5'd01 ; if(counter[3] == 1'b1) sprite_to_Draw_next = 5'd02 ; else begin sprite_to_Draw_next = 5'd03 ; end counter_in = counter +1'b1; // space_on: endcase jman_X_Pos_in = jman_X_Pos + jman_X_Motion; jman_Y_Pos_in = jman_Y_Pos + jman_Y_Motion; sprite_to_Draw_next =last_sprite; end endmodule